CMU 15-112 Summer 2020: Fundamentals of Programming and Computer Science
Collab 6 (Due Thu 28-May, at 11:59pm)
- This assignment is COLLABORATIVE. This means you may work with your week's collaboration group within the course collaboration boundaries. See the syllabus for details.
- To start:
- Create a folder named 'collab6'
- Download all three of these files into that folder:
- collab6.py
- basic_graphics.py
- cs112_m20_unit6_linter.py
- Edit collab6.py using VSCode
- When you are ready, submit collab6.py to Autolab. For this hw, you may submit up to 10 times, but only your last submission counts.
- Do not use sets, dictionaries, or recursion in this assignment.
- Do not hardcode the test cases in your solutions.
- NOTE: Make sure all graphics code (including the tkinter import) goes below the ignore_rest line.
- NOTE: This homework is going to be graded for style. Make sure that you adhere to the style guide when you're working on this HW, and double check your file against the style guide before you submit to Autolab!
- drawStar(canvas, centerX, centerY, diameter, numPoints, color) [35 pts] [manually graded]
Write the function drawStar which takes a canvas and the star's center coordinates, diameter, number of points, and color, and produces a star based on that specification. To draw a star, we need to identify where to place each of the inner and outer points, then draw them all together as a polygon.
The outer points of the star should be evenly placed on a circle based on the specified diameter, with the first point at a 90 degree angle. The inner points should then be placed on a circle 3/8 the size of the first circle, halfway between the pairs of outer points. (We use this ratio to make a nice-looking five-pointed star. Actually, the best inner circle would be about 38.2% the size of the outer circle; a little trigonometry and problem-solving will tell you why! But 3/8 is close enough.) An example of how these circles work is shown below.
Hint: It may be useful to know that canvas.create_polygon
can take in a list of coordinates as its first parameter.
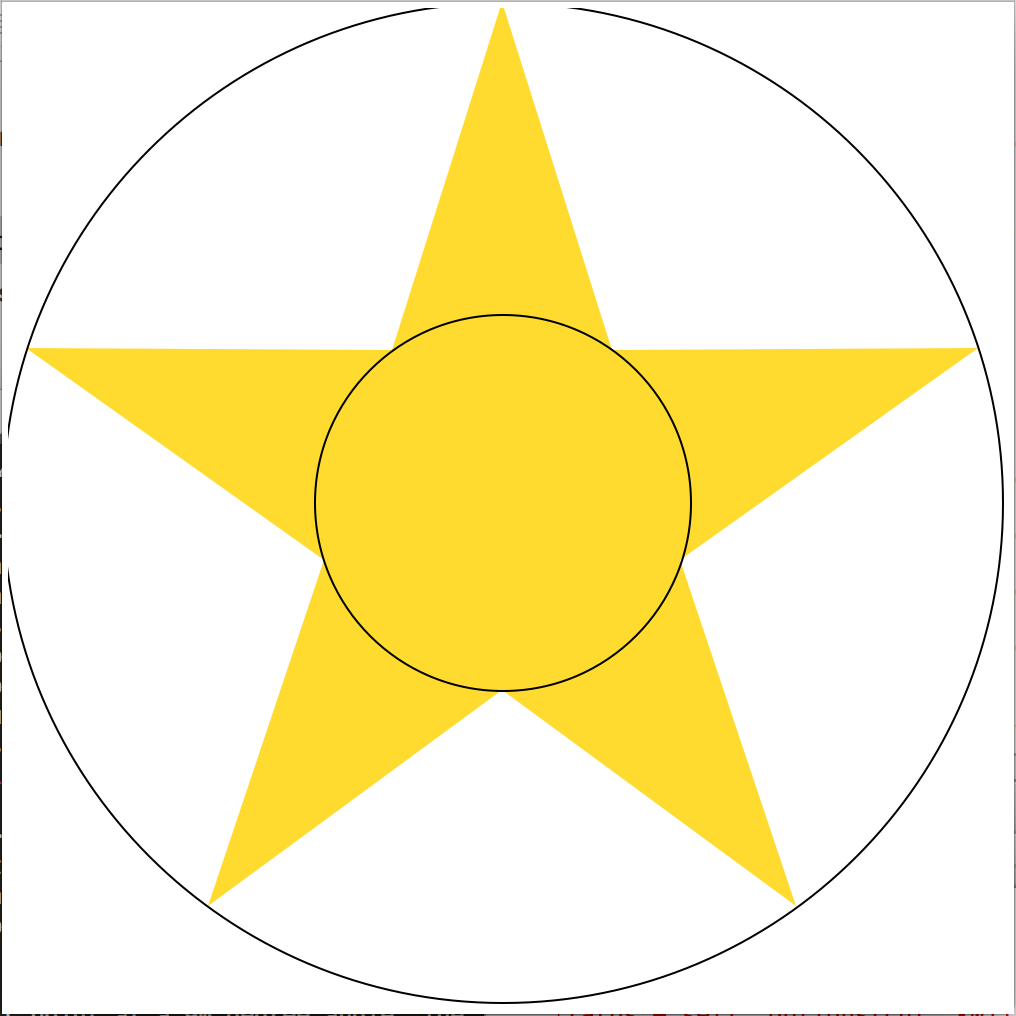
For example, this call:
drawStar(canvas, 250, 250, 500, 5, "gold")
produces this result:
And this call:
drawStar(canvas, 300, 400, 100, 4, "blue")
produces this result:
And if we add a few more points:
drawStar(canvas, 300, 200, 300, 9, "red")
we get this result:
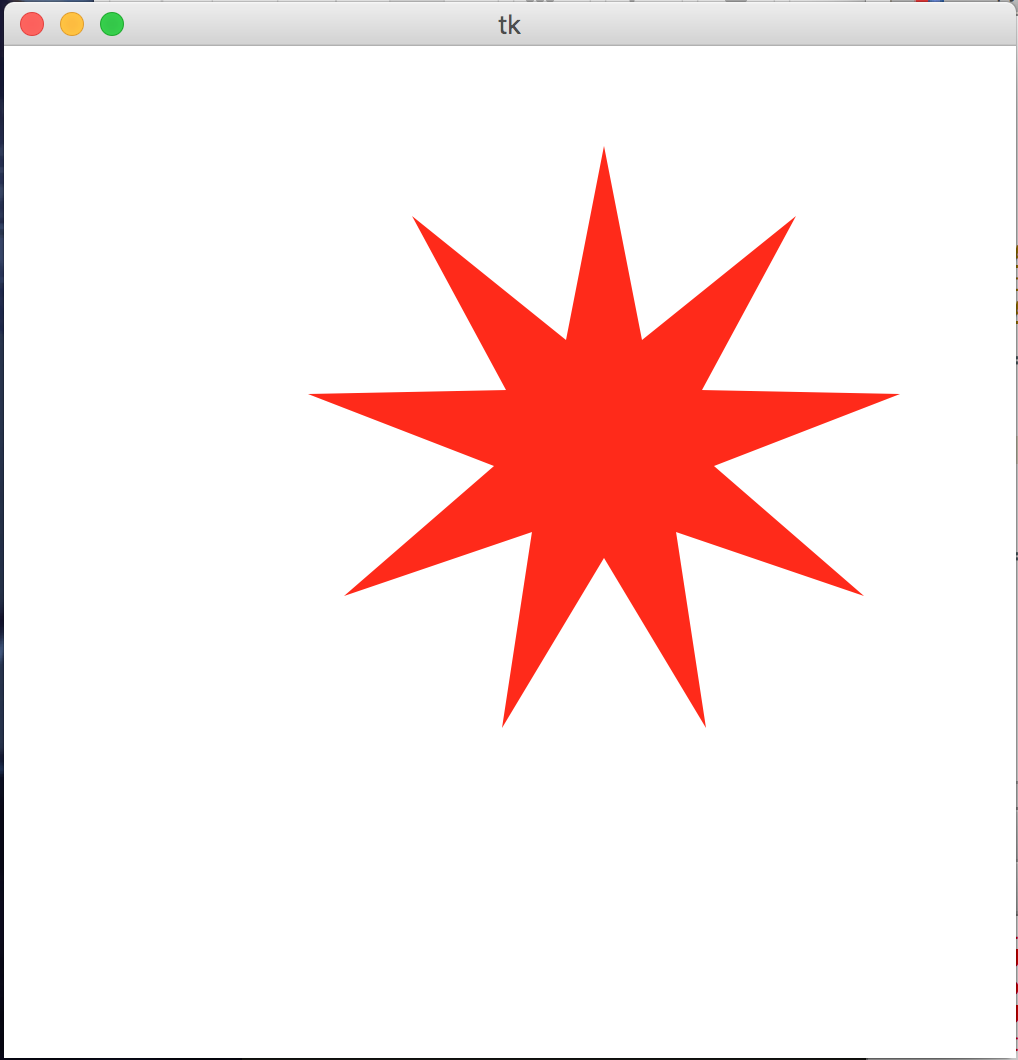
- drawFlagOfPuertoRico(canvas, width, height) [10 pts] [manually graded]
Using drawStar, write the function drawFlagOfPuertoRico(canvas, width, height) which draws the Flag of Puerto Rico on the given canvas. You are guaranteed that the proportions of the width and height will be correct (so width will be 1.5 times the height). As is usual with graphics, your image does not need to be pixel-perfect identical to ours, but it should be fairly similar. Here are a few flags of different proportions:
drawFlagOfPuertoRico(canvas, 600, 400)
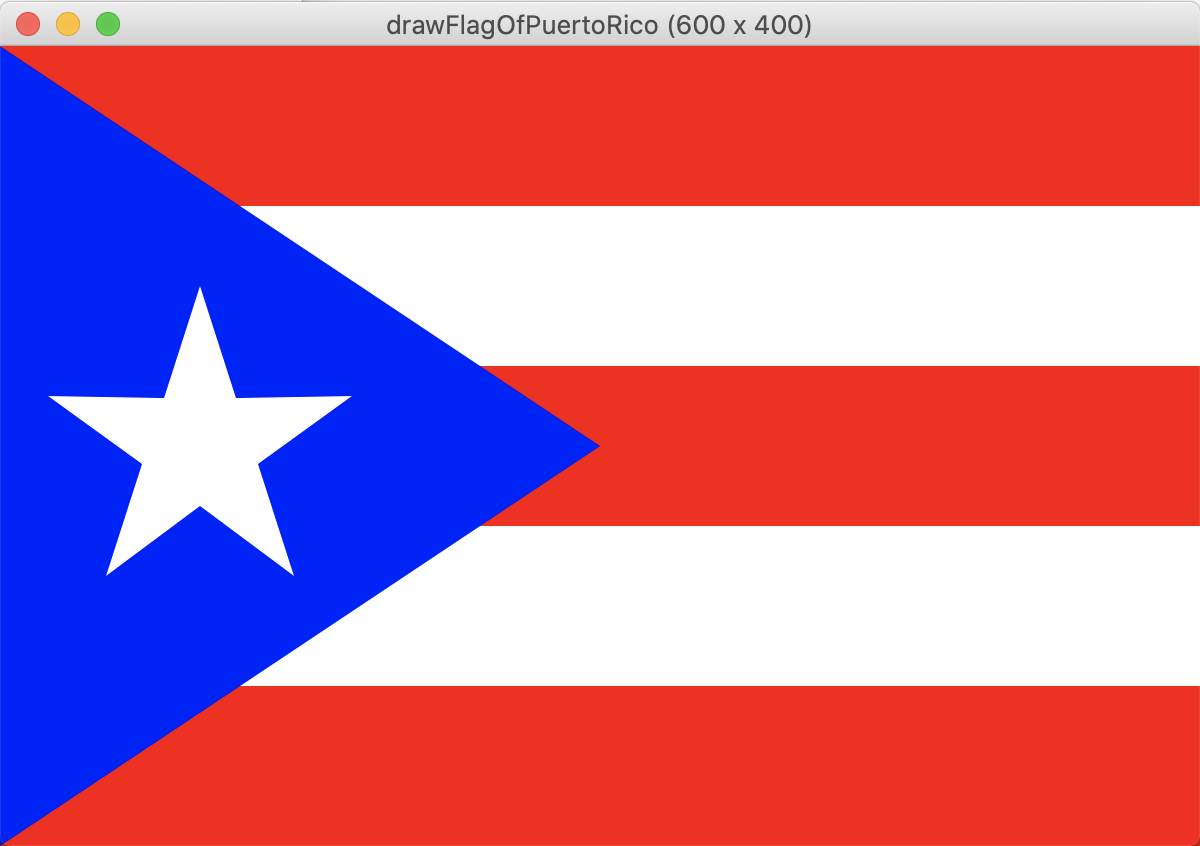
drawFlagOfPuertoRico(canvas, 150, 100)
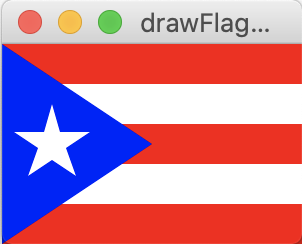
drawFlagOfPuertoRico(canvas, 300, 200)
